728x90
반응형
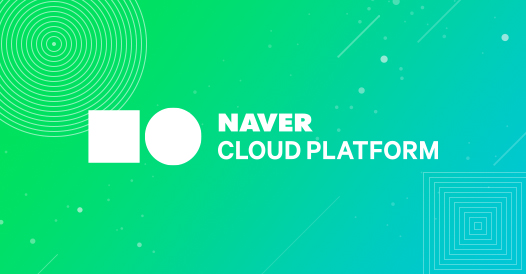
1. 들어가며
- 📌nCloud 통해서 서비스되고 있는 프로젝트에 클라우드 메일 전송 서비스를 개발하면서 정리한 글
- 네이버 클라우드는 개인/일반 기업용과 공공기관용이 따로 있으며 가이드 내용이 상이할 수 있습니다.
2. Cloud Outbound Mailer 사전 준비
2.1 JAVA SDK 다운로드
- 지원하는 API 별로 SDK 제공되고 있어 확인 후 다운로드 [바로가기]
- Cloud Outbound Mailer 제공하는 SDK 다운로드 (공공기관용이 따로 있으니 확인 후 다운로드 바랍니다.)
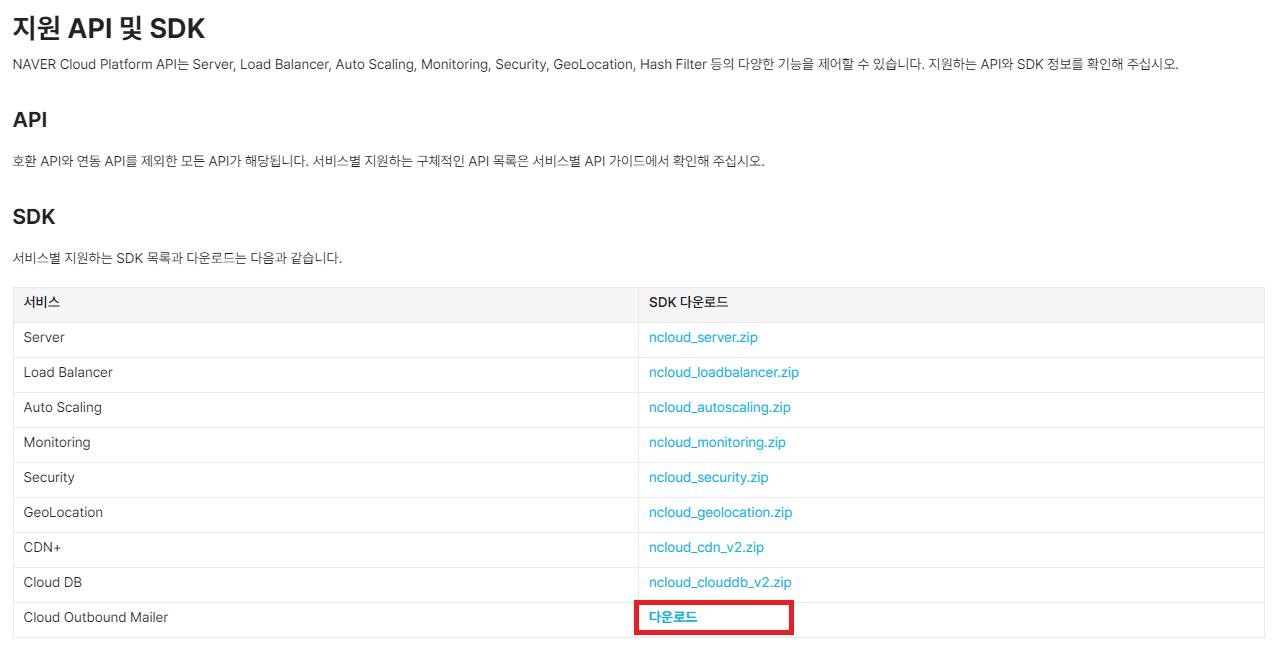
2.2 JAVA SDK 사용하기 위한 준비
- 압출 풀면 나오는 java 소스 또는
nes-client-1.6.1.jar
를 추가 - SDK 사용에 있어 필요한 라이브러리 설치 필자는 maven 프로젝트로
pom.xml
아래 내용을 추가
<!-- https://mvnrepository.com/artifact/junit/junit -->
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
<!-- https://mvnrepository.com/artifact/com.squareup.okhttp3/okhttp -->
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>${okhttp-version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.squareup.okhttp/logging-interceptor -->
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>logging-interceptor</artifactId>
<version>${okhttp-version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.fasterxml.jackson.core/jackson-databind -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>${fasterxml-version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/com.fasterxml.jackson.dataformat/jackson-dataformat-xml -->
<dependency>
<groupId>com.fasterxml.jackson.dataformat</groupId>
<artifactId>jackson-dataformat-xml</artifactId>
<version>${fasterxml-version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/commons-codec/commons-codec -->
<dependency>
<groupId>commons-codec</groupId>
<artifactId>commons-codec</artifactId>
<version>${common-codec-version}</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.commons/commons-lang3 -->
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.7</version>
</dependency>
2.3 credentials.properties 파일 생성
type=iam
apiKey="API Gateway에 설정된 API Key"
accessKey="Sub Account에 설정된 서브계정의 Access Key"
secretKey="Sub Account에 설정된 서브계정의 Secret Key"
3. Cloud Outbound Mailer JAVA 구현 소스
3.1 ApiClient 객체 인증 정보 설정
- 프로퍼티 파일을 이용한 인증
public void setUp() {
apiClient = new ApiClient.ApiClientBuilder()
.addMarshaller(JsonMarshaller.getInstance())
.addMarshaller(XmlMarshaller.getInstance())
.addMarshaller(FormMarshaller.getInstance())
.setCredentials(new PropertiesFileCredentialsProvider("credentials.properties").getCredentials()) // 인증 정보 설정
.setLogging(true)
.build();
api = new V1Api(apiClient);
}
3.2 createMailRequest (메일 발송)
- Email 발송을 요청합니다. 요청 파라미터는 공식 API 문서 확인 바랍니다. [바로가기]
public class CloudOutboundMailer {
public V1Api setUp() {
ApiClient apiClient = new ApiClient.ApiClientBuilder()
.addMarshaller(JsonMarshaller.getInstance())
.addMarshaller(XmlMarshaller.getInstance())
.addMarshaller(FormMarshaller.getInstance())
.setCredentials(new PropertiesFileCredentialsProvider("lib/credentials.properties").getCredentials())
.setLogging(true)
.build();
V1Api apiInstance = new V1Api(apiClient);
return apiInstance;
}
public void createMailRequest() {
V1Api apiInstance = setUp();
List<EmailSendRequestRecipients> esrrList = new ArrayList<EmailSendRequestRecipients>();
EmailSendRequestRecipients emailSendRequestRecipients = new EmailSendRequestRecipients();
emailSendRequestRecipients.setAddress("수신자 메일");
emailSendRequestRecipients.setName("수신자 이름");
emailSendRequestRecipients.setType("R");
esrrList.add(emailSendRequestRecipients);
EmailSendRequest requestBody = new EmailSendRequest();
//requestBody.setTemplateSid({templateID}); 템플릿 사용시 템플릿 번호
//requestBody.attachFileIds("{fileID}"); // createFile를 통해서 생성된 fileId 리스트
requestBody.setRecipients(esrrList);
requestBody.setBody("본문영역");
requestBody.setSenderAddress("발송자 메일");
requestBody.setSenderName("발송자 이름");
requestBody.setTitle("메일 제목");
requestBody.setConfirmAndSend(false);
String X_NCP_LANG = "ko-KR"; // String | 언어 (ko-KR, en-US, zh-CN), default:en-US
System.out.println(requestBody);
try {
// Handler Successful response
ApiResponse<EmailSendResponse> result = apiInstance.mailsPost(requestBody, X_NCP_LANG);
} catch (ApiException e) {
// Handler Failed response
int statusCode = e.getHttpStatusCode();
Map<String, List<String>> responseHeaders = e.getHttpHeaders();
InputStream byteStream = e.getByteStream();
e.printStackTrace();
} catch (SdkException e) {
// Handle exceptions that occurred before communication with the server
e.printStackTrace();
}
}
}
3.3 createFile (메일에 파일 첨부가 필요할 경우)
- 파일을 업로드할 수 있는 기능을 제공합니다. 업로드된 파일의 fileId는 업로드 후 24시간 동안 재사용할 수 있습니다. 24시간이 경과하면 업로드된 파일은 삭제되며 fileId도 유효하지 않게 됩니다. 요청 파라미터는 공식 API 문서 확인 바랍니다. [바로가기]
ublic class CloudOutboundMailer {
public V1Api setUp() {
ApiClient apiClient = new ApiClient.ApiClientBuilder()
.addMarshaller(JsonMarshaller.getInstance())
.addMarshaller(XmlMarshaller.getInstance())
.addMarshaller(FormMarshaller.getInstance())
.setCredentials(new PropertiesFileCredentialsProvider("lib/credentials.properties").getCredentials())
.setLogging(true)
.build();
V1Api apiInstance = new V1Api(apiClient);
return apiInstance;
}
public void createFile() {
V1Api apiInstance = setUp();
File files = new File("업로드할 파일 경로");
String X_NCP_LANG = "ko-KR"; // String | 언어 (ko-KR, en-US, zh-CN), default:en-US
try {
// Handler Successful response
ApiResponse<FileUploadResponse> result = apiInstance.filesPost(files, X_NCP_LANG);
} catch (ApiException e) {
// Handler Failed response
int statusCode = e.getHttpStatusCode();
Map<String, List<String>> responseHeaders = e.getHttpHeaders();
InputStream byteStream = e.getByteStream();
e.printStackTrace();
} catch (SdkException e) {
// Handle exceptions that occurred before communication with the server
e.printStackTrace();
}
}
}
- createFile 통해서 리턴 받는 fileId 사용해 메일에 파일 첨부 하시면 됩니다.
4. 마치며
나머지 궁금한 사항 등은 공식 API 가이드 문서 통해서 확인 바랍니다. [바로가기]
728x90
반응형